In the vast WordPress development landscape, custom post types and taxonomies are powerful tools that allow developers to extend the platform beyond traditional blogging capabilities. While default posts and pages serve a variety of content needs, custom post types and taxonomies enable us to create structured data and organize content in a way that suits a website’s diverse requirements. In this article, we will delve into the world of custom post types and taxonomies, and explore their importance, implementation, and practical applications in WordPress development.
Table of Contents
Understanding custom post types:
In WordPress, post type refers to the different types of content entities that can be created and managed within the system. By default, WordPress comes with built-in post types such as Posts and Pages. However, custom post types allow developers to define their own content structures tailored to specific needs. Whether it’s products, testimonials, portfolio items, events, or any other type of content, custom post types provide the flexibility to organize and display information in a useful way.
Implement custom post types:
Creating custom post types in WordPress involves registering them using PHP code. This process usually occurs within the template’s functions.php file or in a custom plugin. WordPress provides a simple and intuitive API for registering custom post types, allowing developers to define different parameters such as taxonomies, capabilities, rewrite rules, and support features such as custom fields, reviews, and comments. Once registered, custom post types can be managed just like regular posts or pages through the WordPress admin interface.
Let’s create a custom post type named “Books” as an example. Below is the PHP code that you can add to your theme’s functions.php
file or within a custom plugin:
// Step 1: Registering the Custom Post Type
function create_custom_post_type() {
$labels = array(
'name' => _x( 'Books', 'post type general name', 'textdomain' ),
'singular_name' => _x( 'Book', 'post type singular name', 'textdomain' ),
'menu_name' => _x( 'Books', 'admin menu', 'textdomain' ),
'name_admin_bar' => _x( 'Book', 'add new on admin bar', 'textdomain' ),
'add_new' => _x( 'Add New', 'book', 'textdomain' ),
'add_new_item' => __( 'Add New Book', 'textdomain' ),
'new_item' => __( 'New Book', 'textdomain' ),
'edit_item' => __( 'Edit Book', 'textdomain' ),
'view_item' => __( 'View Book', 'textdomain' ),
'all_items' => __( 'All Books', 'textdomain' ),
'search_items' => __( 'Search Books', 'textdomain' ),
'parent_item_colon' => __( 'Parent Books:', 'textdomain' ),
'not_found' => __( 'No books found.', 'textdomain' ),
'not_found_in_trash' => __( 'No books found in Trash.', 'textdomain' )
);
$args = array(
'labels' => $labels,
'description' => __( 'Description.', 'textdomain' ),
'public' => true,
'publicly_queryable' => true,
'show_ui' => true,
'show_in_menu' => true,
'query_var' => true,
'rewrite' => array( 'slug' => 'book' ),
'capability_type' => 'post',
'has_archive' => true,
'hierarchical' => false,
'menu_position' => null,
'supports' => array( 'title', 'editor', 'author', 'thumbnail', 'excerpt', 'comments' ),
'taxonomies' => array( 'category', 'post_tag' ),
);
register_post_type( 'book', $args );
}
add_action( 'init', 'create_custom_post_type' );
Now, let’s explain the code step by step:
- Registering the Custom Post Type: In this step, we define a function
create_custom_post_type()
to register our custom post type using theregister_post_type()
function. Within the function, we define various parameters to configure our custom post type, such as labels, description, visibility, rewrite rules, capabilities, and supported features. - Labels: Labels are used to provide human-readable names and descriptions for our custom post type in the WordPress admin interface. We use
_x()
for translation readiness and__()
for text translation. - Arguments (Args): These are the parameters passed to
register_post_type()
to define the behavior and appearance of our custom post type. Key arguments include'public'
to make the post type publicly accessible,'supports'
to enable support for specific features like title, editor, author, etc., and'taxonomies'
to specify which taxonomies the post type should support. - If you want Custom taxonomy, comment the
'taxonomies'
line in args to remove default taxonomies the post type support.
To implement this code:
- Copy the provided PHP code into your theme’s
functions.php
file or create a new custom plugin. - Replace
'Books'
,'Book'
, and other textdomain strings with your desired names and translations. - Customize the arguments (
$args
) array according to your specific requirements. You can adjust labels, rewrite rules, supported features, and taxonomies as needed. - Save the file and visit your WordPress admin dashboard. You should now see a new menu item labeled “Books,” and you can start adding and managing books as you would with regular posts.
Utilizing Taxonomies for Organization:
Categories in WordPress act as classification systems that help organize content into meaningful groups or categories. While categories and tags are the default labels for posts, custom labels can be created to categorize custom post types or even regular posts in a more organized way. For example, a “Genre” label can be linked to a custom post type “Movies” to categorize movies based on their genres, such as action, comedy, drama, etc. Categories provide a powerful mechanism for filtering, sorting, and retrieving content based on specific criteria.
Let’s register a custom taxonomy named “Genres” for our “Books” custom post type. Here’s the PHP code to achieve that:
// Step 1: Registering the Custom Taxonomy
function create_custom_taxonomy() {
$labels = array(
'name' => _x( 'Genres', 'taxonomy general name', 'textdomain' ),
'singular_name' => _x( 'Genre', 'taxonomy singular name', 'textdomain' ),
'search_items' => __( 'Search Genres', 'textdomain' ),
'popular_items' => __( 'Popular Genres', 'textdomain' ),
'all_items' => __( 'All Genres', 'textdomain' ),
'parent_item' => null,
'parent_item_colon' => null,
'edit_item' => __( 'Edit Genre', 'textdomain' ),
'update_item' => __( 'Update Genre', 'textdomain' ),
'add_new_item' => __( 'Add New Genre', 'textdomain' ),
'new_item_name' => __( 'New Genre Name', 'textdomain' ),
'separate_items_with_commas' => __( 'Separate genres with commas', 'textdomain' ),
'add_or_remove_items' => __( 'Add or remove genres', 'textdomain' ),
'choose_from_most_used' => __( 'Choose from the most used genres', 'textdomain' ),
'not_found' => __( 'No genres found.', 'textdomain' ),
'menu_name' => __( 'Genres', 'textdomain' ),
);
$args = array(
'hierarchical' => true,
'labels' => $labels,
'show_ui' => true,
'show_admin_column' => true,
'query_var' => true,
'rewrite' => array( 'slug' => 'genre' ),
);
register_taxonomy( 'genre', 'book', $args );
}
add_action( 'init', 'create_custom_taxonomy' );
Now, let’s explain the code step by step:
- Registering the Custom Taxonomy: We define a function
create_custom_taxonomy()
to register our custom taxonomy using theregister_taxonomy()
function. Within the function, we define various parameters to configure our taxonomy, such as labels, hierarchical structure, visibility, and rewrite rules. - Labels: Similar to custom post types, labels are used to provide human-readable names and descriptions for our custom taxonomy in the WordPress admin interface. We use
_x()
for translation readiness and__()
for text translation. - Arguments (Args): These are the parameters passed to
register_taxonomy()
to define the behavior and appearance of our custom taxonomy. Key arguments include'hierarchical'
to specify whether the taxonomy should have parent-child relationships (like categories) or not (like tags),'labels'
to define labels for various taxonomy elements, and'rewrite'
to specify the URL structure for taxonomy archives.
To implement this code:
- Copy the provided PHP code into your theme’s
functions.php
file or create a new custom plugin. - Replace
'Genres'
,'Genre'
, and other textdomain strings with your desired names and translations. - Customize the arguments (
$args
) array according to your specific requirements. You can adjust labels, hierarchical structure, and rewrite rules as needed. - Save the file, and your custom taxonomy “Genres” should now be registered and associated with your “Books” custom post type.
With this code, you’ll have a new taxonomy “Genres” available in the WordPress admin interface, allowing you to categorize your “Books” custom post type by different genres.
Full Code
function create_custom_post_type() {
$labels = array(
'name' => _x( 'Books', 'post type general name', 'textdomain' ),
'singular_name' => _x( 'Book', 'post type singular name', 'textdomain' ),
'menu_name' => _x( 'Books', 'admin menu', 'textdomain' ),
'name_admin_bar' => _x( 'Book', 'add new on admin bar', 'textdomain' ),
'add_new' => _x( 'Add New', 'book', 'textdomain' ),
'add_new_item' => __( 'Add New Book', 'textdomain' ),
'new_item' => __( 'New Book', 'textdomain' ),
'edit_item' => __( 'Edit Book', 'textdomain' ),
'view_item' => __( 'View Book', 'textdomain' ),
'all_items' => __( 'All Books', 'textdomain' ),
'search_items' => __( 'Search Books', 'textdomain' ),
'parent_item_colon' => __( 'Parent Books:', 'textdomain' ),
'not_found' => __( 'No books found.', 'textdomain' ),
'not_found_in_trash' => __( 'No books found in Trash.', 'textdomain' )
);
$args = array(
'labels' => $labels,
'description' => __( 'Description.', 'textdomain' ),
'public' => true,
'publicly_queryable' => true,
'show_ui' => true,
'show_in_menu' => true,
'query_var' => true,
'rewrite' => array( 'slug' => 'book' ),
'capability_type' => 'post',
'has_archive' => true,
'hierarchical' => false,
'menu_position' => null,
'supports' => array( 'title', 'editor', 'author', 'thumbnail', 'excerpt', 'comments' ),
//'taxonomies' => array( 'category', 'post_tag' ),
);
register_post_type( 'book', $args );
}
add_action( 'init', 'create_custom_post_type' );
function create_custom_taxonomy() {
$labels = array(
'name' => _x( 'Genres', 'taxonomy general name', 'textdomain' ),
'singular_name' => _x( 'Genre', 'taxonomy singular name', 'textdomain' ),
'search_items' => __( 'Search Genres', 'textdomain' ),
'popular_items' => __( 'Popular Genres', 'textdomain' ),
'all_items' => __( 'All Genres', 'textdomain' ),
'parent_item' => null,
'parent_item_colon' => null,
'edit_item' => __( 'Edit Genre', 'textdomain' ),
'update_item' => __( 'Update Genre', 'textdomain' ),
'add_new_item' => __( 'Add New Genre', 'textdomain' ),
'new_item_name' => __( 'New Genre Name', 'textdomain' ),
'separate_items_with_commas' => __( 'Separate genres with commas', 'textdomain' ),
'add_or_remove_items' => __( 'Add or remove genres', 'textdomain' ),
'choose_from_most_used' => __( 'Choose from the most used genres', 'textdomain' ),
'not_found' => __( 'No genres found.', 'textdomain' ),
'menu_name' => __( 'Genres', 'textdomain' ),
);
$args = array(
'hierarchical' => true,
'labels' => $labels,
'show_ui' => true,
'show_admin_column' => true,
'query_var' => true,
'rewrite' => array( 'slug' => 'genre' ),
);
register_taxonomy( 'genre', 'book', $args );
}
add_action( 'init', 'create_custom_taxonomy' );
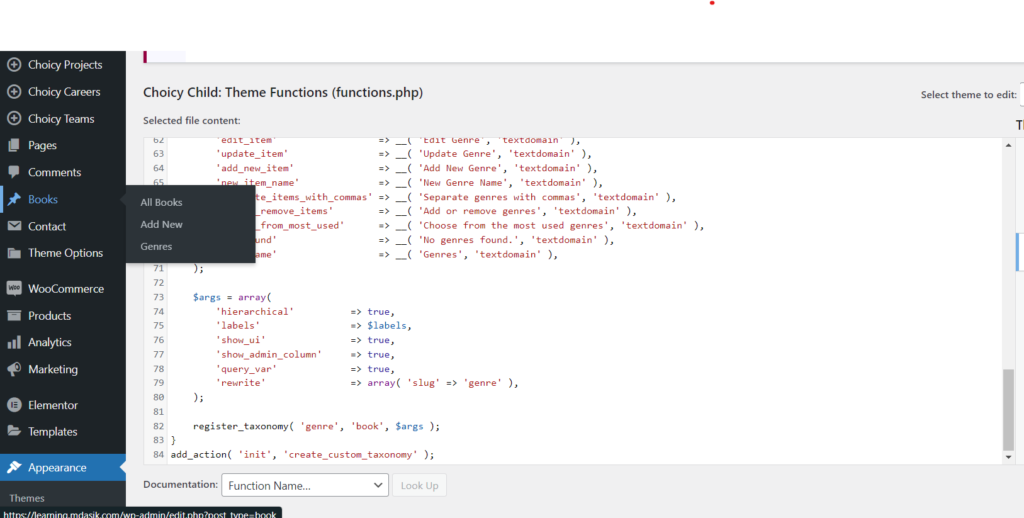
Practical Applications:
Custom post types and taxonomies find myriad applications across various types of WordPress websites. For instance:
- Portfolio Websites: Custom post types can be used to showcase portfolio items, while custom taxonomies can categorize them by project type, industry, or client.
- Real Estate Listings: A custom post type “Properties” can be created to list real estate listings, with custom taxonomies for property types, locations, and amenities.
- Event Management: Custom post types for events can be complemented by taxonomies like event categories, venues, and event organizers for efficient event organization and browsing.
- Product Catalogs: E-commerce websites can employ custom post types for products, with taxonomies for product categories, brands, sizes, and colors.
Conclusion:
Custom post types and taxonomies empower WordPress developers to mold the platform into virtually any type of website, from simple blogs to complex web applications. By harnessing the flexibility and extensibility offered by these features, developers can create structured and organized content systems tailored to the specific needs of clients and users. Whether you’re building a portfolio website, an e-commerce store, or an event management platform, custom post types and taxonomies are indispensable tools in your WordPress development arsenal, unlocking endless possibilities for content organization and presentation.
I hope this article helped you to learn How to create custom post types in wordpress. If you have any doubts or problem with the code, comment below to find the solutions. Also share this blog if you find this useful.
Want to build professional website for your Business or Store, Get a free quote here
Click here to get Premium Plugins and Themes at rs.249. Get 20% Off on your first order “WELCOME20”
[…] our series on mastering custom post types in WordPress! In our previous installments, we explored creating and implementing custom post types, focusing specifically on our “Book” custom post type (CPT). Now, it’s time to […]
[…] on your WordPress website. If you haven’t created one yet, check out our detailed guide on how to create a custom post type in WordPress using code. Make sure you record the important details like post type slug and associated custom […]